Abenity implementation into WordPress

Abenity is a platform that offers deals and discounts to a certain group of users that you have in your Abenity account, but they need to sign into the platform and they offer a SSO way to login and register your users automatically.
In this time I will show how you can configure and make the implementation for Abenity library into a WordPress site using a custom short-code for Abenity links.
- Get the API key from https://backoffice.abenity.com/account/info/api
- Generate your ssh public key:
ssh-keygen -t rsa -b 2048 -C "your_email@example.com"
When you finished the execution of the code it should generates a new file called /home/your_user/.ssh/id_rsa.pub. You need to copy the content code into your Abenity account https://backoffice.abenity.com/account/info/api
cat /home/your_user/.ssh/id_rsa.pub
After you copied the public key from the file you can save the configuration

After that your setup is complete and you can implement the Abenity request API, in our case we use the PHP wrapper https://github.com/Abenity/abenity-php
In order to accomplish that we need to install composer if we didn’t install it, just follow the composer installation documentation here: https://getcomposer.org/download/
And then we need to create a new file in the WordPress root directory, I’m supposing that you are using the command line console
touch composer.json
Then add the require library in the file (you can use your prefer text editor, vim or nano to open the file):
{
"require": {
"abenity/abenity-php": "^2"
}
}
Then you can install the library you need to run:
composer install
In this moment we have the Abenity wrapper installed in our vendor folder and we can continue with the implementation but before it we need to obtain our private key from the file /home/your_user/.ssh/id_rsa
cat /home/your_user/.ssh/id_rsa
We can copy the code in another file in order to continue the implementation we use this key in the parameter $privatekey in our implementation
We will create a new file called abenity.php
touch abenity.php
And we use this example code to make the implementation for the file
<?php
$get_params = $_GET;
// Include autoloader (from Composer)
require 'vendor/autoload.php';
// Define Abenity API Credentials. Replace these with your values.
define('ABENITY_API_USERNAME', 'your_API_USERNAME');
define('ABENITY_API_PASSWORD', 'your_API_PASSWORD');
define('ABENITY_API_KEY', 'your_API_KEY');
// Create new Abenity object
$abenity = new \Abenity\ApiClient(ABENITY_API_USERNAME, ABENITY_API_PASSWORD, ABENITY_API_KEY);
// Define your private key, probably read in from a file.
$privatekey = ''; // HERE YOU NEED TO COPY THE PRIVATE KEY THAT WAS GENERATED IN /home/your_user/.ssh/id_rsa
// Set member profile
$member = array(
'creation_time' => date('c'),
'salt' => rand(0,100000),
'send_welcome_email' => 1,
'client_user_id' => $get_params['id'],
'email' => $get_params['email'],
'firstname' => $get_params['first_name'],
'lastname' => $get_params['last_name'],
'zip' => $get_params['zip'],
'country' => 'US'
);
// Attempt to SSO a member
$abenity_response = $abenity->ssoMember($member, $privatekey);
if( isset($abenity_response->status) ){
if( $abenity_response->status == 'ok' ){
$abenity_url = $abenity_response->data->token_url;
$abenity_url_exploded = explode('https://your_subdomain.abenity.com', $get_params['abenity_link']);
if (isset($abenity_url_exploded[1])) {
$url = $abenity_url . '&page_request=' .$abenity_url_exploded[1];
header('Location: '.$url);
} else {
echo 'Error in url exploding';
}
}else{
// Handle $abenity_response->error ...
var_dump($abenity_response->error);
}
}
To finish this part we will create the short-code custom file to do the implementation for the Abenity short-code, we need to go in the console to our current theme folder for example:
cd /var/www/vhosts/abenity/httpdocs/wp-content/themes/current_theme_name
And we will create a new file called custom-shortcodes.php
touch custom-shortcodes.php
We add this code in that file:
<?php
function generate_abenity_link( $atts = [], $content = null, $tag = '' ) {
// normalize attribute keys, lowercase
$atts = array_change_key_case( (array) $atts, CASE_LOWER );
$user = wp_get_current_user();
$email = $user->data->user_email;
$user_id = $user->ID;
$first_name = get_user_meta($user_id, 'first_name', true);
$last_name = get_user_meta($user_id, 'last_name', true);
$zip = get_user_meta($user_id, 'zip', true);
// override default attributes with user attributes
$abenity_atts = shortcode_atts(
array(
'link' => 'some default link to an Abenity deal',
), $atts, $tag
);
$abenity_link = $abenity_atts['link'];
$params = [
'id' => $user_id,
'abenity_link' => $abenity_link,
'email' => $email,
'first_name' => $first_name,
'last_name' => $last_name,
'zip' => $zip
];
$get_params = http_build_query($params);
$url = get_site_url().'/abenity.php?'.$get_params;
return '<div class="div_abenity"><p><a href="'.$url.'" target="_blank" class="button_abenity">Claim Your Benefit</a></p></div>';
}
add_shortcode('abenity-link', 'generate_abenity_link');
We finish this part after the file was saved and will continue inside our WordPress dashboard
On this way we will load the custom short-codes file using the functions.php file, on our browser we go to the theme editor: https://our_wp_url/wp-admin/theme-editor.php
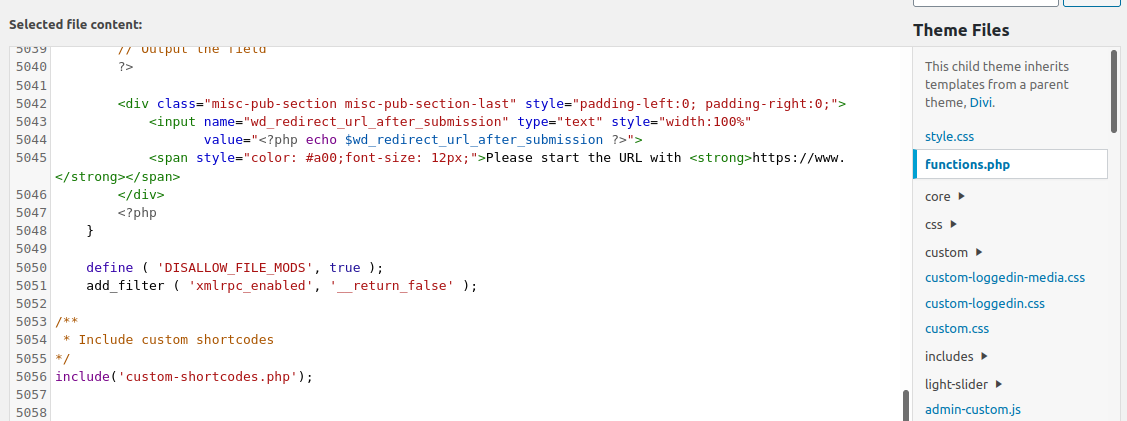
We should add the next code in the last line inside the functions.php file:
/**
* Include custom shortcodes
*/
include('custom-shortcodes.php');
Then we upload the file using the button in the bottom part of the screen
With this, we finished the implementation and we can use the short-code opening a new post and we will add the short-code on this way:
[abenity-link link="https://your_subdomain.abenity.com/discounts/offer/604:41189"]
For the explanation of the link parameter, it is a parameter to generate the Abenity link with a specific deal, that will be opened in a new window after the user clicked the Abenity link.
Well it is the end of this post, I hope this will be useful and if you have any questions please let me know in the commentaries.
Best regards.
Comments ()